Foreword
The purpose of this page is to document all the known tricks of
the NES Mega Man games so far.
Because of the nature of this site, we concentrate here on tricks
that are nearly difficult to play in real play, but are useful
in the making of tool-assisted speedruns. For most of the tricks,
a
frame advance feature in an emulator is a definite must-have.
Note: Rockman is the original (Japanese) name of Mega Man.
Although we have a separate
Glossary page, there are some terms used
on this page that may require explaining. Use this list as a reference.
- Blinking, to blink
When Mega Man or an enemy boss is shot, they’ll blink for a while. During this blinking, no shots will harm them (invulnerability).
- Block
- A square of 16×16 pixels. All levels in Mega Man games are divided in blocks. It is a handy unit of measure of length and dimension.
- Ejection
- When an object is inside a wall, games usually attempt to eject them from the wall. This ejection appears as zipping.
- Frame
- The smallest unit of time in tool-assisted movies.
One refresh period of the screen.
Typically, gaming systems update the screen 50 or 60 times per second. The unit of updates is called frame.
- Lag
- Slowing of the game due to many on-screen objects.
Explained in more detail later in this document.
- Pixel
- Picture element.
The smallest unit of a digital bitmap image; a block is a square of 16×16 pixels.
The NES display area is exactly 256 pixels wide.
- Powerup
- A bonus item, such as an energy pill or an extra life. Acquired by destroying enemies. Subject to luck manipulation.
- Rockman
- Mega Man’s Japanese name is Rockman. He is Rock and she is Roll.
To the people of USA, he was introduced as Mega Man when the first game of the series was imported to USA.
- Teleportation animation
In Mega Man games, Mega Man undergoes a teleportation animation every time he enters a scene or recovers from a pause. During this animation, he’s invulnerable to enemies.
- Wrapping
When the screen edges are connected.
When the bottom side of screen is the closest thing to the top of the screen, or the left side of the screen is the closest thing to the right side of the screen.
- Zipping, to zip
Super fast horizontal movement through the level.
In Mega Man games, when an object is inside a wall, the game attempts to eject it from the wall by zipping it at a speed of 16 pixels/frame until the object finds a hole to exit the wall form. If there is lag, the speed is slower.
In this example image, power-ups that are born inside a wall will be ejected through the wall by zipping.
TODO: New tricks have been found in MegaMan 4 and 5, and this list of tricks, as well as the above statement, need to be updated by someone knowledgeable to reflect that.
Generic techniques
These are tehniques that are common in a lot of games.
Some of them are mentioned at the
Common Tricks page,
but it’s worth listing them here too.
Luck manipulation
Randomness in a game is actually a numeric variable that is affected by
different things in different games. It might be how you move, the timing,
how many shots you shoot, which enemies appear on screen, and so on.
Varying these factors and testing different possibilities (using rerecording)
may enable you to accomplish the most desirable goal.
The likelihoods of each type of drop are:
Type | MM1 (random seeds) | MM2 (random seeds)
|
---|
extra life | 1/128 (63) | 1/128 (62)
|
---|
big weapon refill | 2/128 (5F and 60) | 5/128 (58–5C)
|
---|
big energy refill | 2/128 (61 and 62) | 4/128 (5D–60)
|
---|
small weapon refill | 15/128 (41–4F) | 25/128 (30–48)
|
---|
small energy refill | 15/128 (50–5E) | 15/128 (49–57)
|
---|
bonus pearl | 69/128 (0C–40 and 70–7F) | does not exist
|
---|
nothing | 24/128 (00–0B and 64–6F) | 78/128 (00–2F, 61 and 63–7F)
|
---|
[E] | does not exist | code exists but is unreachable
|
---|
But with luck manipulation, you can get any of those if you try hard enough.
Rockman 1
; This piece of code is executed at every frame. It's invoked by the NMI routine
; even when the game is lagging. The variable $0D is a temporary variable
; used by the game for various purposes. When the screen scrolls, its value
; is 0x03 and otherwise it's 0x78 + number of onscreen sprites, or something
; like that. It is purposely made hard to predict.
RandomUpdate: ;at $D574
lda $0D
eor RandomSeed ;at memory address $46
adc FrameCounter ;at memory address $23
lsr a
sta RandomSeed
; This function is the frontend to randomness calculation.
; Input:
; A = max value
; Output:
; A = random number (smaller than input was).
; Modifies:
; carry flag
; $40
; This function gives the same value for every invokation during
; the same frame. It is used by almost everything in the game.
RandomFunc: ;at $C5A0
sta $40
lda RandomSeed
sec
loop:
sbc $40
bcs loop
adc $40
rts
As an example, here's how the game determines which bonus to give
when an enemy is killed:
CreateBonus: ;at $05BF13
lda #100
jsr RandomFunc
ldy #$3B
ldx #5
loop:
cmp BonusProbabilityTable,x
bcc endloop
iny
dex
bpl loop
endloop:
cpy #$3B ; 3B=no item, 3C=pearl, 3D=small wpn, and so on
beq noitem
; Spawn the bonus drop
tya
jsr CreateEnemy
...
noitem:
...
BonusProbabilityTable:
.byt 99, 97, 95, 80, 65, 12
; Probabilities for:
; 1UP (1%), Big Energy (2%), Big Weapon (2%),
; Small Energy (15)%, Small Weapon (15%), Score Pearl (53%)
Rockman 2
; This piece of code is executed at every frame. It's invoked by the NMI routine
; even when the game is lagging. The variable $480 is the fractional part
; of Megaman's X position. When $480 wraps around a full cycle, Megaman's
; X position will be incremented by 1 pixel. This variable is only
; influenced by Megaman's movements.
RandomUpdate: ;at $D0C6
lda $0480
eor RandomSeed ;at memory address $4A
adc FrameCounter ;at memory address $1C
lsr a
sta RandomSeed
; This function is a generic math function (it performs 8-bit division),
; but it is also used as the frontend to randomness calculation.
; Input:
; $01 = divident
; $02 = divisor
; Output:
; $03 = result
; $04 = remainder (modulo)
; Modifies:
; Y
; carry flag
;
; It is used as a randomness function by copying the random seed ($4A)
; into $01 and setting $02 as the max value.
DivMod: ;at $C84E
lda #0
sta $03
sta $04
lda $01
ora $02
bne nonzero
sta $03
rts
nonzero:
ldy #8
loop:
asl $03
rol $01
rol $04
sec
lda $04
sbc $02
bcc skip
sta $04
inc $03
skip:
dey
bne loop
rts
Optimal refills
The robots you destroy will give different bonuses depending on randomness.
Big powerups are rare, so getting them might require hundreds
of retries at worst case.
Extra lifes are the rarest.
Enemy actions
The actions of the enemies may depend on your movements or on the randomness.
Falling faster
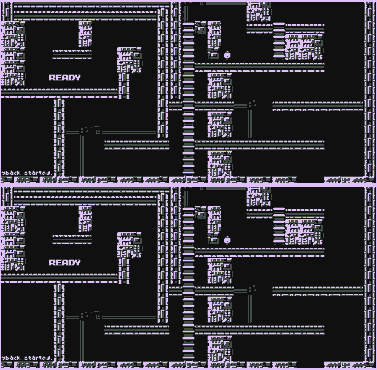
This is a generic trick that is usable in other games besides the Mega Man series.
When you start falling, your movement accelerates slowly.
The longer you fall, the faster you fall.
When you need to fall over an edge, you can fall faster
by jumping high as much before the edge as possible.
This trick only applies to games where jumping is not slower than some other type
of horizontal motion (such as sliding).
Thus, it does not apply to Mega Man 3, but it does apply to Power Blade and Mega Man 2.
In the image pair on the right, you can see that although the difference
in individual actions is small whether jumping or walking, the differences
accumulate quickly and become significant by the end.
Falling even faster (MM1)
In Mega Man 1, there's a third way which is even faster: Falling from a magnet beam.
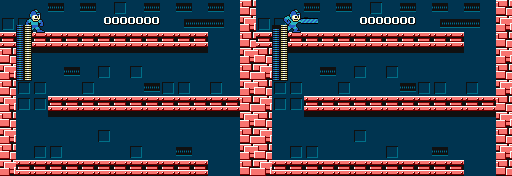
This is because the y speed is increased while standing on the beam, with the beam overriding y movement in order to prevent falling.
Using the fastest method of movement in situation
Jumping is faster than climbing
Jumping is a considerably faster way to elevate than climbing.
Because of that reason, it is worthwhile to use lifts (magnet beam in Mega Man 1)
to enable jumping instead of climbing.
In Mega Man 3, climbing was made somewhat faster, but it’s still faster to jump
than to climb.
Sliding is faster than walking (MM3+)
Falling is faster than climbing
It’s almost never a good idea to climb down a ladder. Instead, if you absolutely
must, grab the ladder for 1 frame, and immediately release it (by jumping).
You’ll fall much faster than you would climb.
You should also try to combine this technique with
the “grabbing the ladder early” technique.
However, in Mega Man 6, you might land on an invisible platform (see below).
Walking is faster than jumping (MM1, MM2)
In Mega Man 1 and Mega Man 2,
Mega Man walks precisely at speed of 1.375 pix/frame. (See
Rockman Technical Data.)
However, when falling or jumping, he progresses only 1.3125 pix/frame.
This is unaffected by the jump start timing or the jump height.
Because of this reason, it is wise to make the jumps as small and rare as possible.
Generic ladder tricks
Grabbing the ladder early (horizontally)
In many platform games, you don’t need to be exactly positioned to
grab a ladder. You can stand about 10 pixels beside the ladder and when
you press up (or down), you’ll immediately grab the ladder.
In Mega Man games, this means that by walking or jumping past a ladder
you can grab the ladder for 1 frame and immediately release it in order
to gain extra movement very quickly. Walking across the ladder would take
about 12 frames, but by grabbing it from distance and releasing it you
can shorten it to about 8 frames.
If you intend to climb the ladder, it’s always wise to grab it as
early as possible.
Avoiding lag
Computer systems run at a set speed, and there’s only so much calculation
they can do in ~0.0167 seconds (the duration of one
frame).
If there are too many objects on screen, or simply too hard calculations
to make, the game won’t be able to make it in 1 frame, and it uses more
frames to do that.
This appears as lag. During lag, your character will move at half
or third of the normal speed. Objects may also blink a lot.
Obviously, it is highly undesirable in a TAS.
To avoid lag, you should try to shoot enemies before they accumulate,
or to avoid shooting when there are already many objects on screen.
Sometimes you can completely delete the enemy by scrolling it out of the
screen (for example, face temporarily left when it appears and immediately
walk to right to scroll it out of visibility).
In Mega Man 1, during zipping (explained later), you can pause+unpause
to create a few frames of completely lag-free movement - since many of
the expensive calculations (such as collision checks) are not done
during the teleportation animation.
Note that static objects (details which are merely part of the level layout)
usually don’t accumulate to lag.
Subpixel position optimization
Rockman moves at subpixel precision. As indicated at
GameResources/NES/Rockman/Data, his horizontal velocity when walking is 01.60 (hex) in Rockman 1-2 and 01.4C (hex) in Rockman 3-4.
Whenever you start moving from a full halt, it is advantageous to choose your subpixel position so that it's in the right direction.
- When you stand at X=100, and you want to move to the left, your X position should be the smallest possible value of 100, that is: 100.000.
- When you stand at X=100, and you want to move to the right, your X position should be largerst possible value of 100, that is: 100.999.
In Rockman 1 for example, you can do this by pushing against the obstacle that prevents your movement. The subpixel position fluctuates, although the integer part is kept constant. Keep pushing until the subpixel position hits an optimal value. When the obstacle clears, you can start moving, and you gain half a pixel of movement by average compared to random case.
- In Rockman 1-2, the possible X fractions are: .0, .0625, .125, .1875, .25, .3125, .375, .4375, .5, .5625, .625, .6875, .75, .8125, .875 and .9375.
- I.e. N/256 for N=0…255 step 16
- In Rockman 3-4, the possible X fractions are: .0, .015625, .03125, .046875, .0625, .078125, .09375, .109375, .125, .140625, .15625, .171875, .1875, .203125, .21875, .234375, .25, .265625, .28125, .296875, .3125, .328125, .34375, .359375, .375, .390625, .40625, .421875, .4375, .453125, .46875, .484375, .5, .515625, .53125, .546875, .5625, .578125, .59375, .609375, .625, .640625, .65625, .671875, .6875, .703125, .71875, .734375, .75, .765625, .78125, .796875, .8125, .828125, .84375, .859375, .875, .890625, .90625, .921875, .9375, .953125, .96875 and .984375.
- I.e. N/256 for N=0…255 step 4
This technique can be applied to other games as well.
Pause tricks
Freeze shots (MM1)
TODO: Add a GIF animation of Gutsman fight, demonstrating how Gutsman blinks while pause and eventually stops blinking.
In Mega Man 1, when you pause using the select button, enemies continue blinking
and may exhaust their invulnerability period during that pause. When you unpause,
the enemies will take a new hit from the shots that are on screen.
Note: Some enemies blink longer than others.
Save vertical time (MM1)
In Mega Man 1, Mega Man continues to move vertically (if he’s performing a jump
or falling) during the “teleportation animation” of the unpausing period. If
you switch weapons at a time when you are jumping upwards or falling down,
you’ll gain time compared to switching weapons at a time when you’re only
trying to move horizontally.
In the images on the right, you can see some differences in timing.
The images are, from left to right:
- Switching weapons while falling (fastest, because useful motion happens during the mandatory wait of the weapon switch)
- Switching weapons at useless moment, but using the “falling faster” trick
- Switching weapons at useless moment, without the “falling faster” trick
Reset acceleration (MM2)
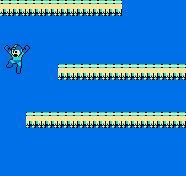
In Mega Man 2, pausing stops Mega Man’s motion. It also resets the acceleration.
This is useful when you want to do extra wide jumps or to fall slower. Just
pause and unpause, and your falling speed resets to 0.
This is a good thing to know if you are a clumsy player and you think Mega Man
is falling too fast for you to handle.
It can also be used to fall into a wall in the Bubbleman level for a nice shortcut
(see abusing the screen breaker).
Remember that to zip, you must be well inside the wall (not too near the left edge),
and that you need to face (not walking, just looking) left.
(Explained later in this document.)
Move through monsters unharmed (MM1, MM2)

In teleportation animation (the animation that occurs when you unpause),
Mega Man is invulnerable. If you move through an enemy or an enemy moves
through you in that mode, you’ll not receive damage.
Note: In Mega Man 1, this technique doesn’t allow passing through spikes.
Regaining motion fast when damaged (MM1)
Normally, when Mega Man collides with an enemy, he’ll lose his motion for
a while, bouncing back a bit. But if you pause+unpause at the moment the
damage is received, the horizontal movement will be cancelled entirely
and you’ll regain control as soon as the pause is over.
Ladder tricks
Grabbing the bottom of the ladder (MM1, MM2)
If you grab a ladder from its very bottommost position (so that it looks
like Mega Man is not actually touching the ladder), and then try to climb
up, Mega Man suddenly elevates by about 24 pixels (his height).
For this reason, it’s sometimes better to not jump as high as possible
when you’re attempting to grab a hanging ladder.
Use this trick to save time in climbing.
Grabbing the top of the ladder too high (MM1, MM2)
If you try to grab a ladder very close to its top (too high to climb it),
Mega Man suddenly elevates by about 24 pixels and ends up in the air above
the ladder.
The same effect also happens if you press up+down at the same time
at the top of a ladder.
If there is a ceiling close to the ladder, you can end up inside
the wall/ceiling this way.
By pressing up+down you can do this trick at every ladder in the game,
but even if you are unable to press up+down, there are approaches.
- In Mega Man 1, place a magnet beam below the top of the ladder. Stand on it, jump and press up.
- In Mega Man 2, place an Item-3 below the top of the ladder. Stand on it, jump and press up.
Applications
Demonstrated in the following illustration:
- Crossing low (32 pixels) tunnels fast
- Entering/passing through a wall
- Walk across a ladder faster
In this artificial illustration using the Mega Man 1 engine,
the following happens:
- At the first ladder, player presses up when he’s already almost above the ladder.
- At the next three ladders, player presses up+down. The third sequence goes through a wall without a problem.
- At the fourth ladder, the player presses up+down in order to do a “horizontal early ladder grab” without climbing, saving a miniscule amount of walking time.
- At the fifth ladder, the player does both the “grabbing the ladder early horizontally” and the “grabbing the bottom of the ladder” trick simultaneously. It is noticeably faster than to jump later & higher, when the space is 3 blocks tall.
Shooting while climbing (MM1)
TODO: Add animation (maybe two animations side by side)
Normally, in Mega Man 1, when you shoot while climbing a ladder, Mega Man
will stop climbing for a second, apparently aiming or looking at the shot.
However, if you jump immediately after shooting, and grab the ladder immediately
after jumping, you can reduce the delay from ~60 frames to about 2 frames
and climb immediately again without losing your position.
Ladder fidget (MM2)
The shooting-and-climbing tech from Mega Man 1 was fixed in Mega Man 2; Mega Man's throwing animation will persist after grabbing the ladder again. However, it's still possible to shave 2 or 3 frames off of a shooting climb via ladder fidget.
To do a ladder fidget, simply drop off and grab back onto the ladder at frame-perfect pace (up, A, wait, up, A, wait...), usually 3 or 4 times. Even though Mega Man physically moves downwards during this process, you'll find that he's higher up after the shooting animation ends. This is due to a double-update bug that decrements the animation timer twice in one frame. This same bug is responsible for the down+B variant of the grabbing the top of the ladder too high tech.
To see how this trick works, grab a ladder from the very top and let go (by pressing jump).
Mega Man will seemingly land on an invisible platform for a fraction of a second.
It is possible to jump from this nonexistent platform, but usually it just slows the
player down because when going down a ladder, Mega Man does not simply fall, but
first lands on the platform. When coming up the ladder, it is not any faster to use
this glitch to make Mega Man jump off from the ladder earlier, unless the ladder
is NOT between two walls (which is surprisingly rare). This glitch does not seem
to appear in Mega Man games 1-5.
Entering ceilings and walls
Why would anyone want to enter walls and ceiling?
Well, that’s because by entering a wall, you can shortcut through it!
It’s a great thing for a TAS.
Methods
The top of the ladder (MM1, MM2)
See grabbing the top of the ladder too high, explained above.
The magnet beam (MM1)
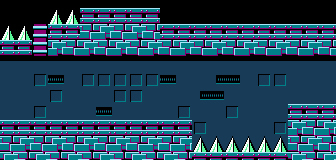
If you stand on a magnet beam that has been placed at a certain distance
down from the closest ceiling, weird things happen.
Basically, the game thinks Mega Man is now inside a wall or ceiling.
Note: The position must be exactly right.
- If it’s too high, Mega Man won’t fit between the ceiling and the beam.
- If it’s too low, nothing special will happen if Mega Man stands on the beam.
The Item-1 (MM2)
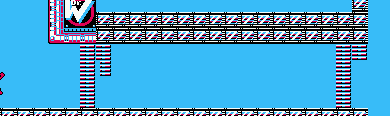
Normally, in Mega Man 2, if you stand on an item-1 that is moving upwards
and you’re about to hit the ceiling, the item-1 disappears to prevent that
from happening.
However, how this is programmed is not obvious. The game does not actually
check whether Mega Man hits a ceiling. Rather, it checks whether the
item-1 is closing towards a ceiling.
So if you place the item-1 so that it is not on the course for hitting a ceiling,
you can put Mega Man standing on the item (with one foot/toe only) and the item will
successfully push Mega Man into the ceiling.
In this image, the player presses right at the right edge of the screen
in order to progress to the next screen without a horizontal wrapping
taking place.
Note: Read the understanding the zipping mechanism chapter to see how you can use
the inside-a-wall condition for your benefit after Mega Man has been placed into a wall.
The Item-3 (MM2)
TODO: Demonstrate item3 with an animation
Item-3 may be used the same way as item-1, but it is limited to
circumstances where the item-3 is actually climbing a wall.
Abusing the screen breaker
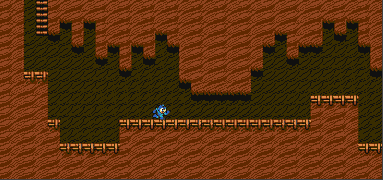
In every Mega Man game, the levels are divided into scenes that are separated
by scrolling transitions. In some circumstances, it’s possible to enter a wall
simply by being in the right position at the edge of the screen.
- MM2: In the Bubbleman level, there’s a position where by using the pause trick to reset acceleration, you can place Mega Man into a wall.
- MM1: In the Fireman level, there is a screen that appears to have a pit in the bottom and a ladder next to it. Jump into the pit (you need velocity), and when the screen scrolls down, Mega Man will be inside a wall.
There are most likely more places you can find.
Dynamically changing levels
- In Mega Man 3 and 4, there are places with stones that drop over Mega Man.
- In all of the Mega Man games, there are rooms with blocks that appear and disappear with a pneumatic sound.
If Mega Man is placed in a place where such block will appear, he’ll be inside a wall.
Frozen fire pillars (MM1)
In the Fireman and Wily1 stages of Mega Man 1, there are pillars of fire
that can be frozen. Normally, they are permeable, but when frozen, they
become solid. Solid material counts as a “wall”.
As shown in this example, this aspect of the game can
be turned into an advantage for creative shortcuts.
| Damage is taken in order to let Mega Man walk through the fire. When the fire becomes ice, Mega Man is inside a wall. After pressing “A”, he will also be inside the floor, which starts the zipping. Just pressing “left” or “right” won’t work, because he would just exit the wall that way (because of proximity to wall left edge). (Note: You can do this in a lot faster manner. Delays were added only for clarity.)
|
---|
Taking damage while sliding near a ceiling (MM3, MM4, MM5, maybe MM6)
In Mega Man 3, a movement called “sliding” was introduced. This movement
allows Mega Man to pass through one-block tall tunnels, when normally
he requires two blocks of height to pass through.
This difference in height comes useful for those who want to abuse it.
With sliding motion, you can get Mega Man’s feet within 16 pixel distance
from the ceiling. Now the only thing to be done is to interrupt the sliding
motion while keeping Mega Man’s height.
This is easiest accomplished by taking damage.
You need two elements:
- A way to bring Mega Man in close proximity to the ceiling. Accomplished by:
- Naturally existing tunnels in the game
- Rush Jet (MM3, MM4, MM6)
- Super Arrow (MM5)
- Balloon (MM4)
- An enemy or shot that approaches Mega Man and delivers damage at the exact moment when the sliding would otherwise stop (floor disappears).
Examples:
| Rockman 3: Rush Jet, 2-block hole and an enemy. Immediately before getting damage, press down and then start.
|
---|
Understanding the zipping mechanism
When Mega Man is inside a wall, even partially, the game wants to eject him.
Horizontal movement
According to current knowledge, all the NES versions have these same rules so far.
In the Rockman Megaworld (Wily Wars) game of Sega Genesis/Megadrive,
consider left and right reversed.
There are two aspects for the ejection to consider.
- The position of Mega Man in pixels, relative to the left edge of the wall. Denoted by
P
.
- The direction Mega Man is facing.
- If
P
< 16, you can walk left and exit the wall this way.
- If
P
< 16, you can face right and the game will eject Mega Man immediately from the wall without delay.
- If
P
>= 16, you can face right and nothing will happen. That is, unless you are at the right edge of the screen, then you will scroll to the next screen. (see below)
- If
P
>= 16, you can face left and zipping will occur: Mega Man will move to the right at 16 pixels/frame.
Exchanging facing and walking
If you want the game to consider you...
- to be walking instead of facing, you can jump and immediately start holding the direction.
- to be facing instead of walking, you need to throw something that prevents walking, such as an Item-1 in Mega Man 2. Or you can wait until Mega Man settles down, and then face the direction.
Jumping
If you press A while standing / zipping inside a wall, Mega Man will try jumping 2 frames later.
- If, at that moment, he is still inside a wall, he’ll move down by 16 pixels for 1 frame and immediately bounce back.
- If, at that moment, he is no longer inside a wall, he’ll jump normally.
However, if there’s an item-1 pushing Mega Man higher into a wall,
jumping may speed up his elevation in the wall.
The top of the screen
See the chapter about vertical wrapping, explained below.
The right side of the screen, aka. horizontal wrapping
See the chapter about horizontal wrapping, explained below.
Screen shaking
In Mega Man 1, the zipping has a tendency to make the screen shake a bit (rough ride?). It happens in Mega Man 2 too, but not as noticeably.
It happens because the PPU (graphics processor) can only handle so much data
during a vrefresh, and if too much data is attempted to be written, it will
affect the scrolling registers. Although it’s a visual glitch, it has no effect
on the game.
The vertical screen wrapping phenomenon
The screen top works differently in Mega Man 1 and Mega Man 2, thus
this section is split. In Mega Man 3-6, none of these techniques work.
Mega Man 1
Shortly: If you go high enough, you can access the bottom of the screen.
Application: Accessing a screen-bottom ladder from top of screen
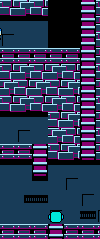
If there is a ladder at the bottom of the screen, you can grab
it from the top of the screen if you can just get there.
Hold
up to grab the ladder.
Note: This is only possible in rooms that have an ‘up’ exit.
The room is not required to have a ‘down’ exit.
TODO: Add screenshot.
If you stand on a magnet beam that has been placed near the screen top,
and there is a ladder directly on below it at the screen bottom, you can
hit the ‘up’ button to grab the ladder, and the screen will scroll
up.
Doing that you may enter a wall.
Application: Using the screen bottom as a ceiling when at the top of screen
If you have used the magnet beam trick to place yourself at a certain position
near the top of the screen, Mega Man’s head will be touching the screen bottom,
and a zipping may occur as long as far there is a solid block at the screen bottom.
Note: In Mega Man 1, the screen bottom must not be lava or other lethal
material -- if it is, Mega Man will simply die upon hitting it.
In Mega Man 2 and the Sega Genesis versions, temporary
invulnerability (blinking) allows to endure spikes.
Special case: Walls at the top of the screen

It is possible to pass through a wall that is at the top of the screen.
To pass through the wall, apply the “use screen bottom as a ceiling” trick,
but before passing through the wall, wait for a while.
Approximately 32 frames of wait is necessary.
If the wait is omitted, Mega Man will die the instant he enters the wall.
[1]
To wait, face right.
To attempt zipping, face left.
[
1] In Rockman 1 and 2, Rockman’s Y speed grows all the time when he’s airborne, even when he’s standing on a beam. (The speed grows 0.25 units per frame, and the maximum Y speed is 12.) The ceiling-zipping only works after Rockman’s Y speed grows beyond 8.0.
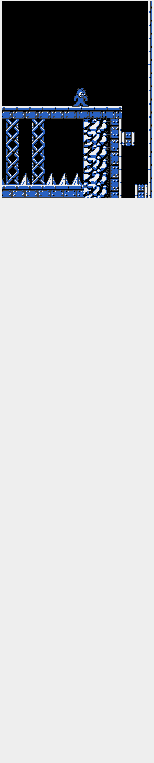
Usually, if you are standing on a magnet beam that is placed near the
top of the screen, and you jump, a death occurs regardless of the contents
of the bottom of the screen.
However, if the screen you’re currently in could scroll down
(nearly always when the screen can not scroll further to the right),
by pressing A the screen will scroll down, and a death does not occur.
Special case: Chaining technique (postponing a jump to next screen)
If you jump on a screen-top beam and
there is no wall on the bottom of the screen
at that position, the jump will be postponed to the next screen and
it will continue upwards after the screen has scrolled!
This is demonstrated in the rightside image.
If you place a magnet beam at the top of the screen immediately after that
(without landing anywhere), you can jump again and the screen will scroll
further down - sooner&faster than if you fell through the whole screen!
Note: The exact pixel position of the beam is very important for the chaining.
Sometimes you may need to adjust the jump timing / position by a few frames
until it works fine.
Mega Man 2
In Mega Man 2 and the Sega Genesis version of Mega Man 1, there is no vertical wrapping.
Instead, if you are positioned very up, you can actually access the next screen.
For example, you can grab an up-going ladder from the area of the next screen.
The horizontal screen wrapping phenomenon
Like the vertical wrapping, also the horizontal wrapping works
differently in each of these games, thus this section is split.
Generally speaking
If, by zipping, you’ll pass the right edge of the screen,
the following thing happens:
You’ll pass through into the next screen.
The next screen may be the room below you, or the room above you, or the
room at the right, depending on the room where you do it.
- If this room has an exit to the right, the next room is on the right.
- If the room has a down exit, the next room is below.
- If this room has no down exit but has an exit to the up, the next room is on the above.
TODO: Verify if this is really independent of the flow of the level.
However, the screen does not change! Mega Man will appear on the left side of the
screen, and all enemies and graphics will stay the same. Only Mega Man himself
knows he is in the next room, because the obstacles he runs into are different
from what appears on the screen.
Note: If the next room is on the right, you can enter the room
properly (without wrapping) by changing the facing to the right
one frame before wrapping.
Now, from this point, the games are different.
Mega Man 1
You can walk left if you wish.
If you walk enough to the left, you’ll be back to the actual visible room.
If you try to face right, you’ll be moved back into the right edge of the visible room!
You can use this technique to jump very fast when the right edge of the
original screen is wall and the left edge of the next screen is not wall.
Mega Man 2
You can continue walking to the left or to the right.
If you walk enough to the left, you’ll be back to the actual visible room.
If you go to the right, you can progress as far as you like, with a certain limitation:
Ladders and doors only work if there is a similar exit in the visible room.
- If you try to climb down a ladder or fall into a pit and the visible room has no down-exit, a death occurs.
- If you fall into a pit and the visible room has a down-exit, you’ll progress to the next or the previous room.
Mega Man 3-6
No wrapping. If you zip into the right edge of the screen, you are stuck,
unless you can somehow get out (with Wire adapter in MM4, for example).
In Mega Man 4-6, in addition to being stuck, Megaman will be killed
unless he’s still blinking.
Miscellaneous tricks
Poking magnet beams through walls (MM1)
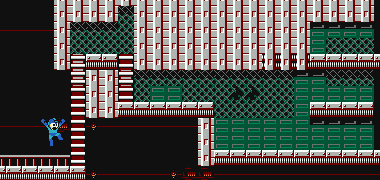
In Mega Man 1, it is normally impossible to put a magnet beam through a wall.
However, the check that prevents doing so, is done very simply: It only checks
whether the last segment of the beam is colliding with a wall. To put a beam
through wall, you only need to grow it somewhere where there isn't wall (often,
facing the opposite direction accomplishes this), and then face towards the wall
when the beam is long enough that its end would be beyond the wall's outer edge.
This is useful when you want to place a beam in a place where there's not enough
room to set it up. In the example shown on the right, the tunnel is too narrow;
Rockman couldn't set up the beam low enough for him to stand on it, if he didn't
poke it through that wall.
Placing beams in the next screen (MM1)
TODO: Add animation.
By utilizing the techique explained above, you can put beams into the next
screen by tunneling them through the right edge of the screen. Next to the right
edge, is the left edge of the next screen (regardless of whether your room is
a vertical or horizontal type).
The beams don't die when screen scrolls, so this might be useful to put the
beam to a place normally impossible to access.
Safe jumping in Gutsman battles (MM1)
When Gutsman jumps, the ground trembles, making Mega Man lose balance
if he stands. However by jumping quickly enough you can jump even
if the ground trembles, keeping you mobile.
In Mega Man 2, there’s a peculiar glitch, that may happen when the game lags.
Rooms, that are normally not supposed to scroll to the up, may provide an up exit.
Rooms that are normally not supposed to scroll to the right, may provide a right exit.
This is possible because in MM1 and MM2, there are actually no concepts of room that is up, down, or right, but instead there’s just next room and previous room.
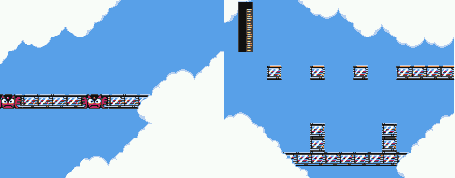
In this example image, the screen is supposed to scroll to the down (next room), but due to lag, a right exit also appears. It also leads to the next room. Both destinations are the same. It is just the next room.
It is also possible to utilize the lag to create an “up” exit, but it requires the presence of a ladder in the bottom of the screen. Such situations usually take you
to the previous room instead of next, and are not much of use in speedruns.
Sliding over short pits (MM3)
Mega Man 3 was the first game of the series to implement a sliding
movement. The implementation has a certain quirk in it: It is sometimes
possible to slide over pits that are two blocks wide. The distance to
the pit edge at slide start decides whether it will work or not.
This trick doesn’t seem to work in Mega Man 4-6.
Cutman's magic scissor (MM1)
In Mega Man 1, the robot master Cutman's AI has a quirk that can be useful
in some ways. It is difficult to explain, but here are the key facts:
- When you escape Cutman's battle, but Cutman manages to throw a scissor, Rockman will begin to jerk up and down erratically, unpredictably, and most likely leading to his death ― in worst case, leading to the game’s reset.
Here's the technical explanation of the trick as it was
used in the tool-assisted movie.
When a boss battle begins, there are only three ways to end it:
- Kill the boss
- Start another boss battle
- Die
When the Cutman battle is entered, and subsequently escaped,
the battle does not end. It goes on. Cutman is still alive.
Momentarily after escaping the room, Cutman throws a scissor.
However, because Cutman is no longer visible, the scissor does not appear. Cutman thinks he has a scissor, but there is no scissor.
This is when things go awry. You see, as long as Cutman thinks he has a scissor, he will use some kind of telekinesis to manipulate the scissor back to his hand (for catching). In other words, the game adjusts the scissor's position every frame to make it meet Cutman's hand.
The method it does that, is that it first looks up the object table, searching for a scissor type object. Then it alter the properties of the found object (the sprite number, and the Y coordinate).
It does the lookup using a common function in the game code.
However, Cutman is so certain that a scissor exists, so the return value of the function is not checked. So when a scissor doesn't really exist, it writes into a random memory address instead. (The actual value returned in an error case is the number of alive objects.) This memory address happens to coincide mostly with Rockman's data. The rest is pretty obvious.
The glitching stops when the Clone robot fight begins, because at that point, the previous boss fight is over and Cutman with his magic scissor powers exists no more.
However, Megaman maintains a weird pose for a few frames, because his object properties (mainly, an action delay counter) were messed up.
Skipping a predefined event (Protoman event) (MM3)
As demonstrated in this image, it’s possible to skip a scene with
Protoman in the Gemini Man stage of Mega Man 3. To do it, start a slide
just before the screen starts scrolling, and when slide continues in
the next screen, jump before the slide stops.
If the slide stops before you jump, you lose control of Mega Man and
you’ll have to wait until Protoman teleports to the room and destroys
the cylinder blocking your way.
There will be some graphics glitches in the rest of the stage when you
do this trick, and most notably the music is gone, but otherwise the
stage is still completable as usual.
Walking on air (MM6)
TODO: Add animation (slow motion).
The fact that Mega Man can stand on a ledge even if a large portion of his body
is standing on thin air is an important part of Mega Man physics, but in MM6 it
is possible to stand completely on air. To do it, just walk over a ledge and you
can see that you are still walking even after the entire Mega Man sprite has
passed the platform. If you stop, you will fall, but if you jump, you gain
extra length. This glitch works best on ice; you can walk a little bit more
than normally because the ice is slippery. The place where this trick is most
useful is at the end of Blizzardman’s level. By utilizing it, it is possible
to avoid falling into a relatively narrow pit with a timebomb in it in the
third to last screen (counting from the boss door).
The basic principle is the same as in the ladder trick: a nonexistent
platform that you can land on and jump from. In addition to all ladders,
there are a few places where these platforms appear.
The first one is at the end of Blizzardman’s level, right before the
boss door, under the timebombs. By utilizing this platform it is
possible to avoid detonating the final time bombs. To perform it, simply
slide in the hole between the time bomb and the icy wall. When falling
down, press right and you will land on the platform. You must jump
off of it quickly, or else you will fall into the pit. The platform does
not occur all the time, and it is very risky to try to use this in normal
gameplay without save states.
The second place where these platforms occur is in Dr. X stage 3, where
there are large rooms filled with some sort of scales. If you land on
one end it is lowered, and the other one rises. Next to each of these
platforms there is an invisible(nonexistent) platform. You must land
on it on a specific position, or else it will not be there. Usually
they just appear when falling down and leaning against the platform.
Again, you must jump off of it quickly if you wish to save your life.
These platforms also appear next to the flipping platforms with spikes under
them (or on top, when they are flipped). There is one of these at the end of
Tomahawkman’s stage (the BEAT route) and one in Dr. X Stage 4.
These platforms also seem to appear in the vicinity of the white
left-to-right flipping platforms, which are found in Windman Stage,
Dr. X Stage 1 and Dr. Wily Stage 1.
The platform usually appears when one jumps on the platform while
it is still in the middle of the flipping process. The player then
finds himself on an invisible platform just under the flipping platform.
You can’t jump off it because the white platform is blocking the way,
so the only option is to fall down. Using this invisible platform is
quite useless.
This glitch has also not been detected in any of the other Mega Man games.
See also
Cheat codes
If you need to use cheat codes for testing, you can
use these shell script to automatically toggle/generate them
for you (in FCEU):
Demonstration movies