So,
I made some modifications to the neatevolve.lua and created some more outputs in the Banner. Also I made the Fitness form bigger and the Save and Load buttons bigger.
Script
http://pastebin.com/drKN5t2F
Must restart to work with new values which get stored. Will not work with your *.state.pool
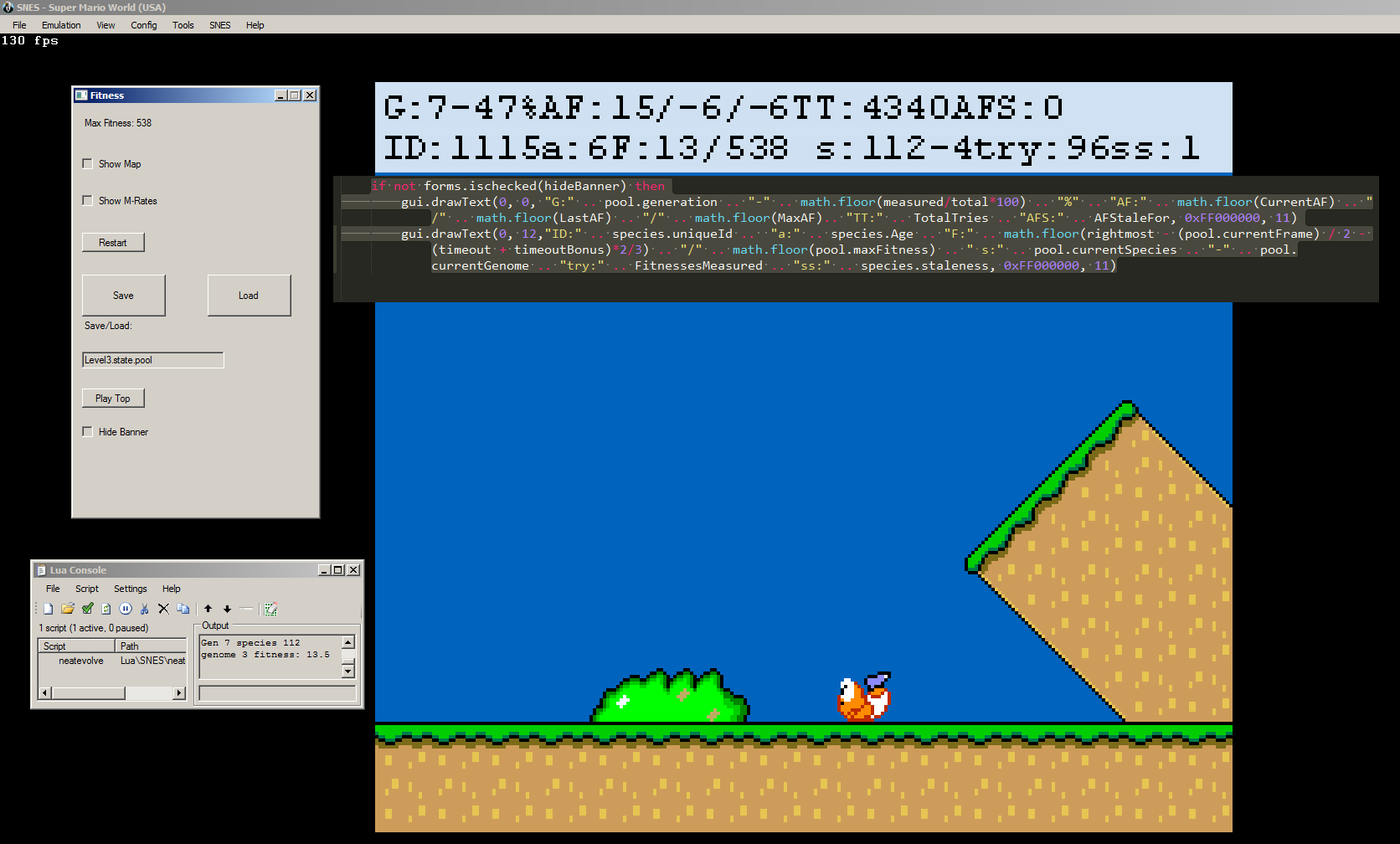
~zoom if you like~, ctrl+
Generation 7, 47% done
G:7-47%
Avarage fitness current generation / Avarage fitness last generation / Max Avarage Fitness
AF: 15/-6/-6
TotalTries that this *state.pool has done
TT:4340
Avarage fitness stales, counts the generations with no new Max Avarage Fitness, here 0 means last Generation hat a new MaxAF
AFS:0
UniqueSpeciecsId, each new Species gets a new id and the UniqueSpeciesId goes +1, 1115 means that this is the 1115th species created in this *.state.pool (it is tied to a specific species)
Id:1115
Age of that species, the amount of Generations they have survived in this *.state.pool
a:6
Fitness of the current run / Maximum Fitness (means best run)
F:13/538
CurrentSpecies - genome (changes each generation, is not tied to a specific species)
s:112-4
The 96th run in this Generation, -- must ranme to run
try:96
1 generation has passed since this species has beaten its own best fitness in this .*state.pool (species.staleness)
ss:1
Must restart to work with new values which get stored. Will not work with your .*state.pool
The code is a mess, feel free to critisize and improve or ask questions.
Also: (solved)
Can I somehow remove the biasCell
local biasCell = {}
biasCell.x = 80
biasCell.y = 110
biasCell.value = network.neurons[Inputs].value
cells[Inputs] = biasCell
without making the program crush? I think MarI/O gets wrong level input and that makes him ultimately less intelligent. Allthough he will probably start running right sooner with the biasCell, it is impacting on his decisionmaking later by giving faulty level information, doesn't it?. Ideas appreciated.
Also:
When suddenly my FPS dropped (from ~150 to ~50 over night) in Windows 7 playing around with Visual Properties of Windows 7 + disabling Windows Fullscreen Hack in Display options in Bizhawk did the trick for me. Something with how Windows displays its windows. The first screenshot shows the options i mean, not sure which did the trick:
http://www.sevenforums.com/tutorials/1908-visual-effects-settings-change.html