Academics! (Level 23)
Algorithm and Part Optimization Walkthrough
The task: take an input string of only red and blue symbols and reverse it.
Unlike for our Robomecha solution, there isn't a clear correct basic algorithm to start with. Our obvious starting points are:
[Algorithm v1.0a]
Loop
1. Read the last symbol.
2. Move it to the front.
End Loop
[Algorithm v1.0b]
Loop
1. Read the first symbol.
2. Move it to the back.
End Loop
v1.0a may be particularly attractive to us because it looks an awful lot like Robomecha, which we've already solved. Let's examine the basic apparatus required for the first step of each algorithm, though. Non destructively read the last symbol:

Non destructively read the first symbol:

Familiarity notwithstanding, it looks like v1.0b might be the simpler approach, both from an algorithmic standpoint and a parts-used standpoint. Let's flesh out the starting point we chose, then.
[Algorithm v2.0]
Loop
1. Read the first symbol.
2. Transcribe the rest of the string.
3. Write the first symbol at the end.
End Loop
In Manufactoria this becomes:
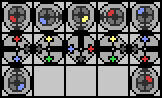
Actually running this, we note a few problems. One, our robot has no way to exit the device, and two, our naive algorithm doesn't actually do what we thought it would do. Successively moving each first symbol to the back of the entire string doesn't reorder the string. We need to move each first symbol to the back of
the portion that hasn't been moved yet. (Or, alternatively, move each first symbol to the front of the portion that
has already been moved.) So we need a marker for both the end of the entire string, and the end of the unprocessed portion of the string. Luckily, Manufactoria gives us another color. Back to the algorithm:
[Algorithm v3.0]
1. Place a G marker. (End of the unprocessed portion.)
2. Place a Y marker. (End of the entire string.)
Loop
3. Read the first symbol.
4. Transcribe until you hit G.
5. Write G.
6. Write the first symbol.
7. Transcribe until you hit Y.
8. Write Y.
End Loop
In Manufactoria:
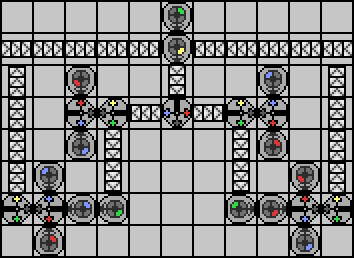
There are more conveyors there than necessary, but hopefully they help to delineate each part of the device as they correspond to steps in our algorithm. Anyway, we're getting quite close now to a device that actually works. Do we have an exit strategy? It turns out we don't need any modifications to exit the device. Once the entire string has been reversed, the G marker indicating the start of the reversed string will in fact be the start of the entire string. That is, the string will be of the form G...Y where the ... in the middle is any sequence of reds and blues. The initial R/B branch in our device that determines the first element of the string will naturally and correctly direct the robot down instead of to either of the colored branches. At that point all we need to do is remove the leading G and terminal Y and be done. Our fully functional device:
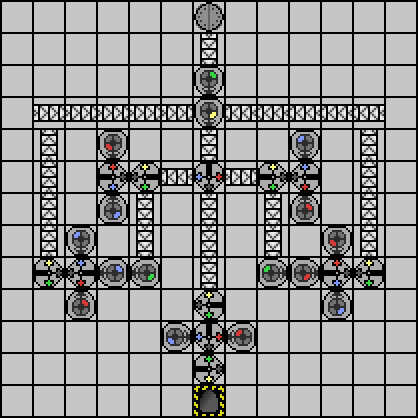
Now let's start optimizing! As always our first step is to look for duplicated components. The main portion of our device has four transcribers, two for each branch. Moreover,
each transcriber has a different exit condition. The first transcription exits on G. The second transcription exits on Y, and never the twain shall meet. We can almost assuredly use both the same transcriber
and the same G/Y branch for both steps! Let's try it:
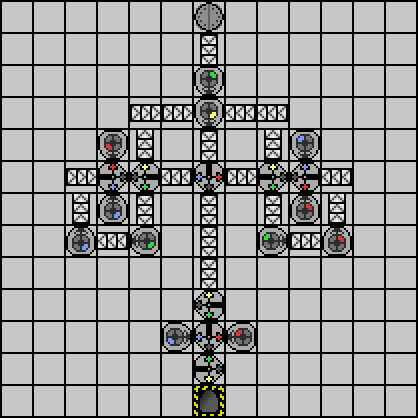
If you've read the Robomecha walkthrough, you should quickly recognize another optimization. We have a situation where we are writing a color, then transcribing the rest of the string. We can do this with fewer parts by simply entering the transcriber through the correct writer. Combine that with the straightforward removal of extraneous conveyors and we are left with a very compact device indeed:
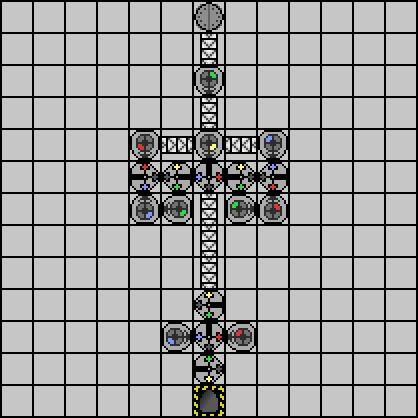
Or if you prefer the level code:
?lvl=23&code=p12:6f3;g12:3f3;c12:2f3;q12:10f0;q12:12f2;p12:11f3;b11:11f2;r13:11f0;c12:7f3;c12:8f3;c12:9f3;y12:5f3;c12:4f3;r10:5f3;p10:6f2;b10:7f1;c11:5f2;q11:6f0;c13:5f0;q13:6f6;b14:5f3;p14:6f0;r14:7f1;g11:7f0;g13:7f2;
And if you wanted to use this entire device as a component in another device, you could trivially mash all the parts together to fit inside a 5x6 box.
Enjoy!