The Lua scripts used on FE6 should be easily adaptable for FE8, since more or less all the GBA games used the same engine. It shouldn't be hard to work out memory locations in FE8 comparable to the ones in FE6. Definitely be useful for some of the more complex enemy phases.
For example there's this script which was created mostly thanks to amaurea:
Download rngdisplay.luaLanguage: lua
function nextrng(r1, r2, r3)
return AND(XOR(SHIFT(r3, 5), SHIFT(r2, -11), SHIFT(r1, -1), SHIFT(r2,15)),0xFFFF)
end
function rngsim(n)
local rngbase=0x03000000
local result = { memory.readword(rngbase+4), memory.readword(rngbase+2), memory.readword(rngbase+0) }
for i = 4, n do
result[i] = nextrng(result[i-3],result[i-2],result[i-1])
end
return result
end
local phit = 0x0203CDAE
local pdmg = 0x0203CDB2
local pcrt = 0x0203920A
local ehit = 0x0203CDAC
local edmg = 0x0203CDB0
local ecrt = 0x0203CDB4
while true do
local nsim = 20
rngs = rngsim(nsim)
for i = 1, nsim do
gui.text(228, 8*(i-1), string.format("%3d", rngs[i]/655))
end
gui.text(210,0,"RNG1:")
gui.text(210,8,"RNG2:")
gui.text(210,16,"RNG3:")
gui.text(194,24,"Next RNs:")
gui.text(0,0,"Player")
gui.text(0,8,"Hit: " .. memory.readbyte(phit))
gui.text(0,16,"Damage: " .. memory.readbyte(pdmg))
gui.text(0,24,"Crit: " .. memory.readbyte(pcrt))
gui.text(0,40,"Enemy")
gui.text(0,48,"Hit: " .. memory.readbyte(ehit))
gui.text(0,56,"Damage: " .. memory.readbyte(edmg))
gui.text(0,64,"Crit: " .. memory.readbyte(ecrt))
emu.frameadvance()
end
This ends up looking like this on screen:
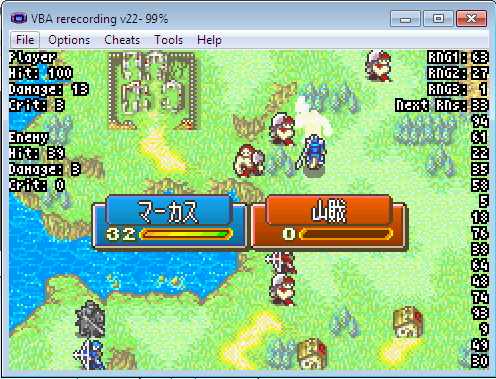
Here, Marcus is performing a crit on the bandit. As you can see, it shows the RNs listed in memory, plus the next 17 RNs on the right side of the screen. It also displays the hit/damage/crit. The addresses in FE8 are different for the combat display, but as you know the RNG is the same so doesn't need changing. It should be pretty easy to find the hit, damage, and crit memory locations and adapt this script for those purposes. One final thing to change is the fact the current RNG value is divided by 655.36 in FE7 and 8, the 655 in this program is a rounding error present in FE6, and needs to be fixed here.
One thing I want to add to this is the active player unit's wexp values. Not sure how to do that yet.